Indentation
In Python, code blocks are identified through the use of indentation. Indentation involves leaving a specific number of spaces before starting to write code. As the number of spaces changes, so does the block to which the code belongs.
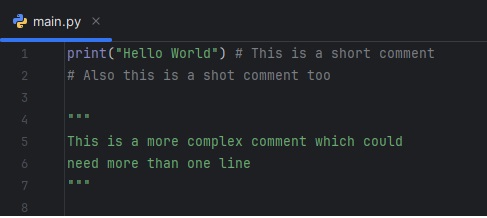
Python doesn’t enforce a fixed number of spaces, but it does require consistency in your choice: once you decide on a certain number of spaces for indentation, you must maintain that throughout the file.
Typically, either a single tab (TAB key) or 4 consecutive spaces are used, with the latter generally recommended by the community.
Comments
As with any programming language, Python allows you to use comments.
Comments are very useful for adding information to the code, making it more understandable to other developers (or even to your future self). A comment is essentially code that is not executed during the program’s runtime.
Single-line comments are introduced with the character #
, followed by a space. For multi-line comments, three pairs of quotes """
are used.
print("Ciao Mondo") # Questo è un commento breve
# Anche questo è un commento breve
"""
Questo è un commento più lungo ed articolato
che può stare su più righe
"""
Variables
Python is a dynamically typed language, meaning that you don’t need to specify the type of a variable when declaring it. The variable’s type is determined automatically during runtime based on the assigned value.
x = 0 # Questa è una variabile con valore iniziale 0
Input and Output
Now that we’ve seen how to declare a variable, let’s see how to assign it a value and how to print its content.
x = 0
y = 0
input("Inserisci il valore di x: ")
input("Inserisci il valore di y: ")
print(f"Hai inserito {x} come valore di x e {y} come valore di y!")
At this link, you’ll find a very simple script that demonstrates how to use what we’ve just learned.
Summary
Now that we understand what Python is, why it’s a powerful and versatile tool, and why learning (at least) the basics can be extremely useful, we’ve also seen how to declare variables, assign values to them, and read their contents. In the next article, we will delve deeper into the various implicit types of variables, explore different data types, and learn how to manipulate them to take full advantage of Python’s capabilities.