In any activity that involves creating something, there is always the possibility of wanting to make changes to your work, only to realize that you’ve made a mistake and can’t go back. And this is precisely where version control systems come to the rescue!
Version Control System
One of the first challenges faced during professional growth in the field of computing is managing code and its changes.
Imagine writing a function, and after hours of work, you decide to modify some parts, only to realize that the new changes not only don’t work but weren’t even necessary. Moreover, the classic CTRL+Z might not be enough to go back far enough.
It’s in situations like this that using a version control tool becomes essential.
A version control system is software that allows you to manage the source code. With a version control system, you can:
- Have multiple versions of the same source code
- Switch between versions
- Track the history of changes and restore the code to a certain point in the past
Among the various version control systems, probably the most famous and widely used is GIT.
Created by Linus Torvalds in 2005, GIT has become one of the essential tools for every developer, right after the IDE.
Installing GIT
To install GIT, simply visit this link and download the version suitable for your operating system.
Main GIT Commands
Although GIT is integrated into almost all major IDEs, which provide convenient graphical interfaces for version control management, GIT was originally designed to be used via the command-line interface.
Below are some useful commands for daily usage via the command line:
- Configure your name: git config –global user.name “NAME”
- View the configured name: git config –global user.name
- Configure your email: git config –global user.email “EMAIL”
- View the configured email: git config –global user.email
- Initialize GIT in the current directory: git init
- View the repository status: git status
If there are already files in the current directory, the command will display a result similar to this:
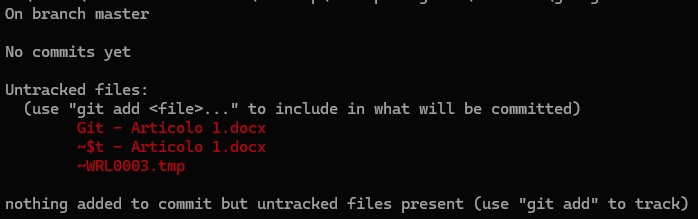
At this point, you can add one or more files to the repository:
- Add a specific file: git add FILE1.EXT FILE2.EXT …
- Add all files in the current directory: git add .
COMMIT
After adding the files to GIT, it’s time to make a commit, which means recording the changes in the repository:
- Commit: git commit -m “COMMENT”
- View the most recent commit: git log
- View the commit in a single line: git log –oneline
At this point, you may realize you forgot to add a file. To fix this, simply add the file and run the following command to amend the previous commit:
- Amend commit: git commit –amend -a -m “COMMENT”
BRANCH
Suppose you want to implement a variant of the code, which develops in parallel to the main version. In these cases, branches are used.
- Create a new branch: git branch “BRANCH_NAME_WITHOUT_SPACES”
- View existing branches: git branch
As mentioned earlier, it is possible to switch between branches. This process is called “switch,” and it can be done by switching to an existing branch or creating a new branch during the switch:
- Switch branches: git switch “BRANCH_NAME”
- Create a new branch and switch: git switch -c “BRANCH_NAME”
- Rename the current branch: git branch -m “NEW_NAME”
- Delete a branch: git branch -d “NAME”
- Merge a branch into the current branch: git merge “BRANCH_NAME”
In previous versions of GIT, the command checkout
was used instead of switch
, and the option -b
instead of -c
.
STASH
Now, suppose you have some changes in progress, but you need to work on another branch. Since it’s good practice not to commit incomplete and untested code, you can save your work in the stash and retrieve it later.
- Save current work: git stash
- Restore from stash: git stash pop
- List stash entries: git stash list
- Restore a specific stash entry: git stash apply stash@{ID}
- Remove a stash entry: git stash drop stash@{ID}
- Clear all stash entries: git stash clear
RESTORE
Another important feature is the ability to restore a file to a specific state, provided the changes have not yet been committed:
- Restore a file to its state in the last commit: git restore FILE-NAME.EXT
- Restore a file to its state at a specific commit: git restore —source BRANCH_CODE FILE_NAME.EXT
If you want to remove a file from staging, you can use:
- git restore —staged FILE_NAME.EXT
RESET & REVERT
If you want to remove a commit and all associated work, there are two options:
- Remove the commit while keeping the local files: git reset BRANCH_CODE
- Remove the commit and the local files: git reset –hard BRANCH_CODE
- Undo committed work by creating a new commit: git revert BRANCH_CODE
REBASE
It is possible to manipulate the commit history to hide, rename, merge, or delete one or more commits. These operations are delicate and should only be performed in exceptional cases:
- Basic command that opens an editor displaying recent commits: git rebase -i HEAD~x (x is the number of commits to display)
- If no changes are needed (default behavior): pick COMMIT_CODE ORIGINAL_MESSAGE
- To edit a commit message: reword COMMIT_CODE NEW_MESSAGE
- To edit a commit: edit COMMIT_CODE NEW_MESSAGE ; at this point, proceed with the following commands before continuing with the rebase:
- git add FILE.EXT FILE.EXT ..
- git commit –amend
- git rebase –continue
- Merge a commit with the previous one (the final message will be that of the previous commit): fixup COMMIT_CODE
- Delete a commit: drop COMMIT_CODE
Suppose you have the following commits to edit:
abcd – Added JWT authentication
efgh – Added refresh token JWT
ilmn – Added README.md file
If you realize that the third commit was unnecessary and there’s a typo in the second commit message, the steps are:
- Open the commit editor: git rebase -i HEAD~3
pick abcd Added JWT authentication
pick efgh Added refresh token JWT
pick ilmn Added README.md file
- Modify the situation as follows:
pick abcd Added JWT authentication
reword efgh Added refresh token JWT
drop ilmn Added README.md file
At this point, after saving the file, Git will ask you to enter a new message for the modified commit. Once you do that, the rebase will be complete.
TAG
A tag is additional information associated with a commit and is generally used to identify a particularly important version, such as a milestone or a version released to production. There are two types of tags: lightweight tags and annotated tags.
- Lightweight tag: git tag v1.0.0
- Annotated tag: git tag -a v1.0.0 -m “TAG MESSAGE”
As with anything, you can list existing tags or delete one or more:
- List tags: git tag
- Delete a tag: git tag -d v1.0.0
Tags can be pushed to the repository either individually or all at once:
- Push a single tag: git push origin v1.0.0
- Push all available tags: git push origin –tags
Conclusions
What we’ve seen here is just a small part of the available commands and options for Git. If you want to delve deeper into the topic, I recommend visiting the official site and consulting the documentation, which you can find here.